5 Great Libraries to Help You Write iOS Apps Faster
Autolayout DSL, animations, keyboard optimization, and more
Originally published on Medium by Zafar Ivaev.
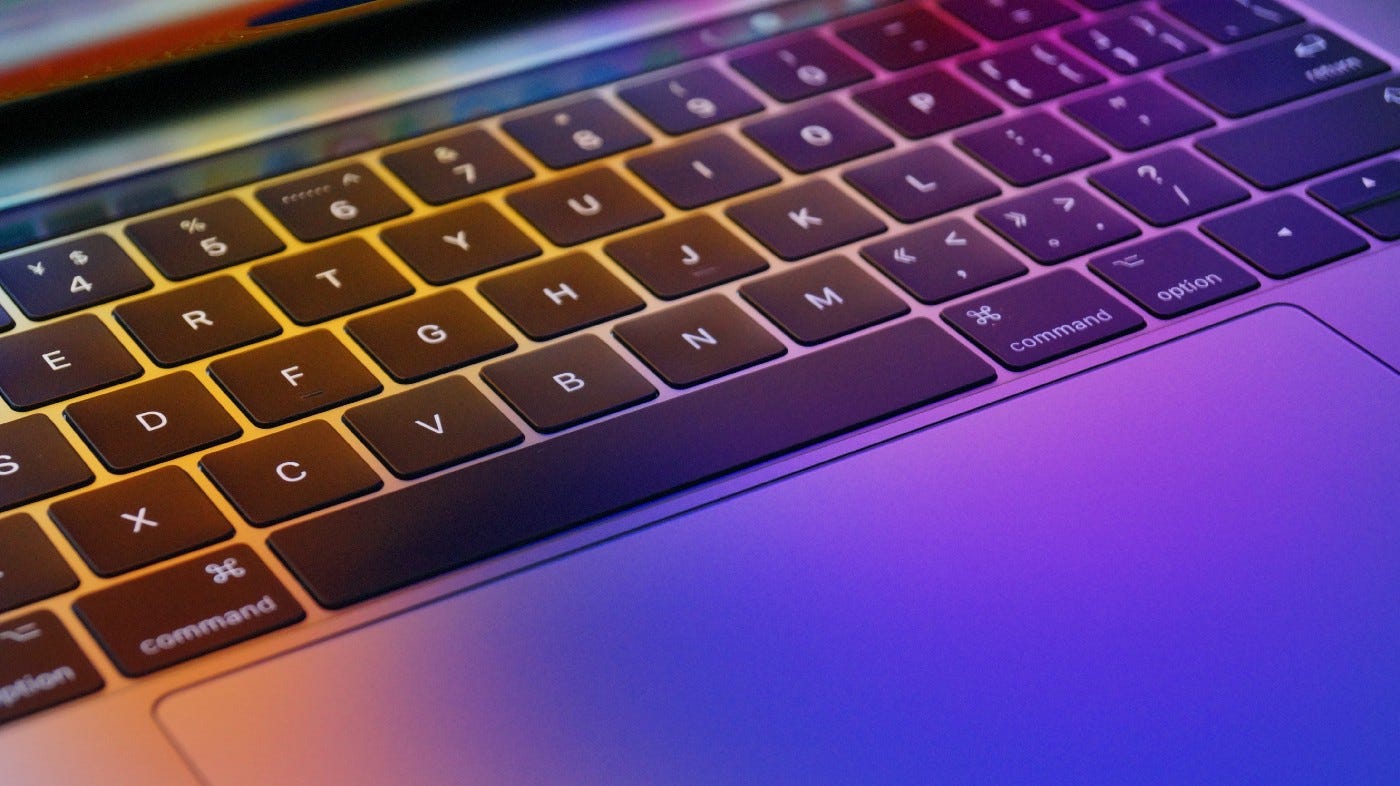
In this article, I’m going to list five great third-party frameworks/libraries that you can import in your app and use to suit your needs.
1. SnapKit — SnapKit helps us create user interfaces programmatically faster.
Here is a simple positioning of a square UIImageView
in the center of its superview:
logoImageView.snp.makeConstraints { (make) in
make.width.height.equalTo(UIScreen.main.bounds.width * 0.5)
make.centerX.centerY.equalToSuperview()
}
As opposed to the standard NSLayoutConstraint
:
NSLayoutConstraint.activate([
logoImageView.widthAnchor.constraint(equalToConstant: UIScreen.main.bounds.width * 0.5),
logoImageView.heightAnchor.constraint(equalToConstant: UIScreen.main.bounds.width * 0.5),
logoImageView.centerXAnchor.constraint(equalTo: self.view.centerXAnchor),
logoImageView.centerYAnchor.constraint(equalTo: self.view.centerYAnchor),
])
2. Lottie — Greatly simplifies handling advanced animations by loading and rendering them in a JSON format.
Thanks to it, developers can easily configure animations like this one:
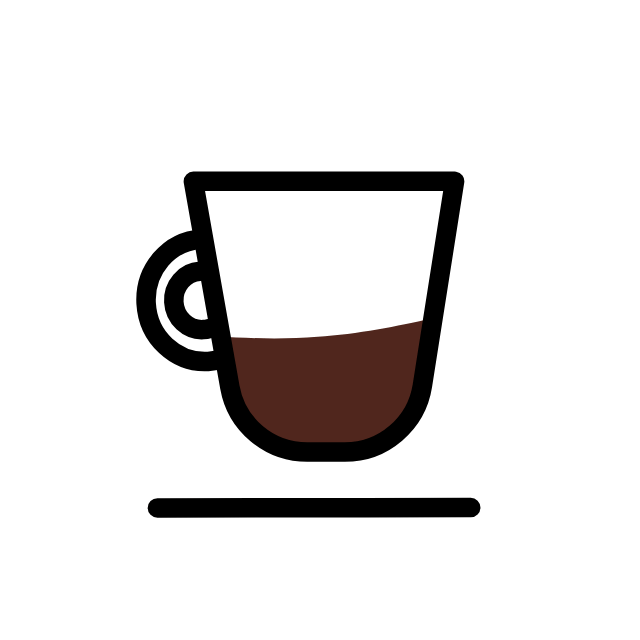
For more, check its GitHub repo.
And the official documentation for iOS/macOS.
3. IQKeyboardManagerSwift
Have you ever had to manually deal with situations where the keyboard overlaps your user interface?
IQKeyboardManagerSwift
is a savior in such cases. All you need to do to forget about handling keyboards is add the following two lines in the AppDelegate
:
import IQKeyboardManagerSwift
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
IQKeyboardManager.shared.enable = true
IQKeyboardManager.shared.enableAutoToolbar = true
return true
}
}
The result now looks like this:
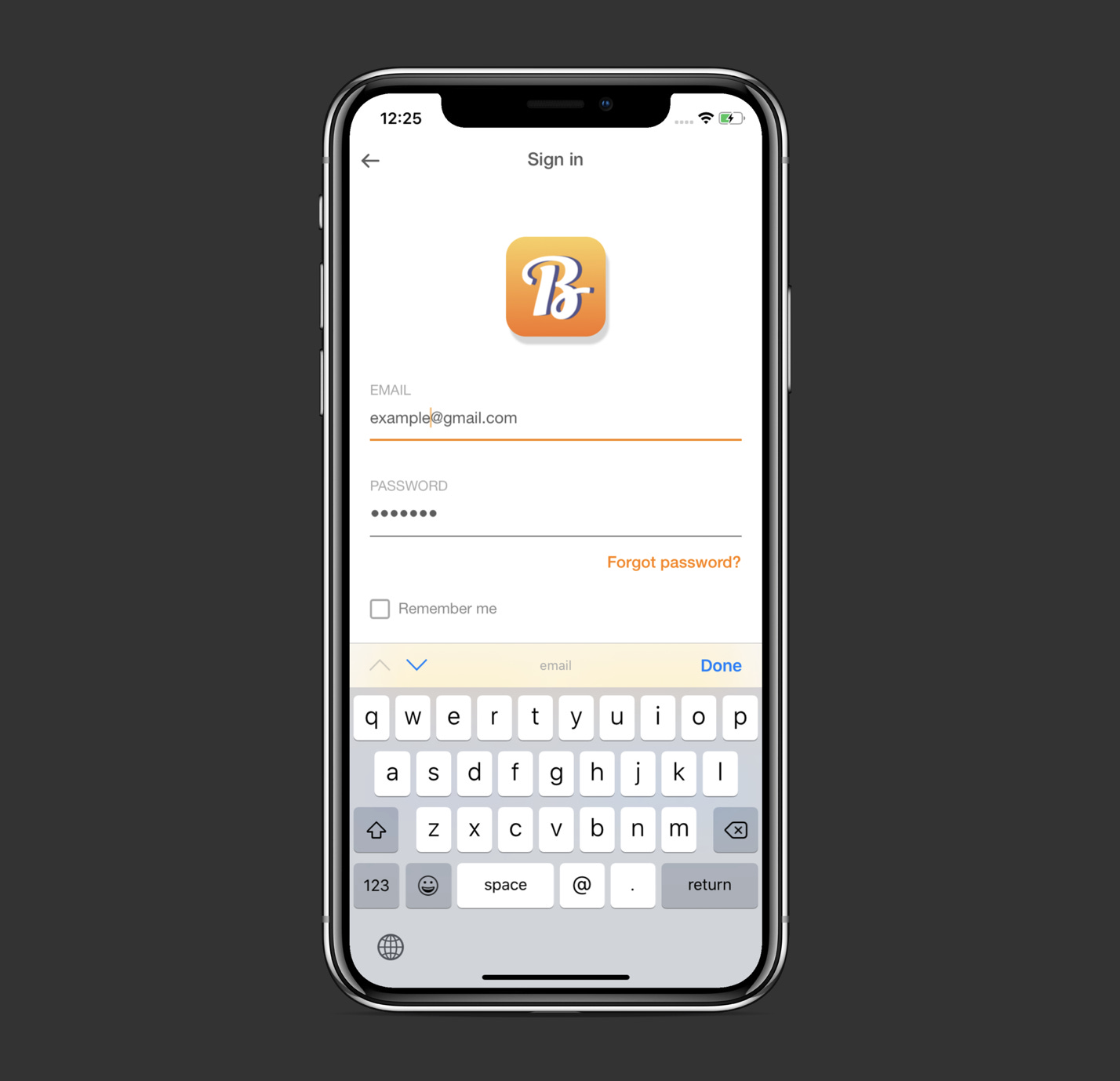
4. PKHUD — Provides great functionality for displaying progress on the screen. It is useful in cases like networking and other time-consuming operations.
Usage is very simple:
Import PKHUD at the top of a file.
Fire loading indicator:
HUD.show(.progress)
orHUD.show(.labeledProgress(title: “Loading”, subtitle: nil))
.Stop loading indicator:
HUD.hide()
.
5. SideMenu — It is common to have a requirement of implementing a side menu (a.k.a. NavigationDrawer
) in an iOS app. The SideMenu
library offers a great solution for this.
Working with it is very simple:
Define the
SideMenuNavigationController
subclass:
Inside your view controller, create and present this subclass:
Note that you specify the content view controller for the side menu as the rootViewController
property.
The SideMenu
library offers many other great perks, like styling customization, presentation type, and others.
Wrapping Up
Like the article? Subscribe to be notified when a new article gets published:
Thanks for reading!